实验三 二叉树的基本操作(建立)及遍历
实验目的
1.学会实现二叉树结点结构和对二叉树的基本操作。
2.通过对二叉树遍历操作的实现,理解二叉树各种操作,学会利用递归方法编写对二叉树等类似递归数据结构进行处理的算法。
实验要求
1.认真阅读和掌握和本实验相关的教材内容。
2.编写完整程序完成下面的实验内容并上机运行。
实验内容
1.编写程序输入二叉树的结点个数和结点值,构造下图所示的二叉树。
2.编写程序,采用中序遍历的递归和非递归算法对此二叉树进行遍历。
提示
从键盘接受输入(先序),以二叉链表作为存储结构,建立二叉树(以先序来建立),
[测试数据]
如输入:ABC##DE#G##F###(其中#表示空格字符)
则输出结果为
先序:ABCDEGF
中序:CBEGDFA
后序:CGEFDBA
层序:ABCDEFG
下面是代码,供参考:
#include #include #define MAX 20 #define NULL 0 typedef char TElemType; typedef int Status; typedef struct BiTNode { TElemType data; struct BiTNode *lchild,*rchild; } BiTNode,*BiTree; Status CreateBiTree(BiTree *T) ///BiTNode T { char ch; ch=getchar(); if(ch=='#')(*T)=NULL;///#代表空指针 else { (*T)=(BiTree)malloc(sizeof(BiTNode)); (*T)->data=ch;///生成根结点 CreateBiTree(&(*T)->lchild);///构造左子树 CreateBiTree(&(*T)->rchild);///构造右子树 } return 1; } ///先序遍历输出 void PreOrder(BiTree T) { if(T) { printf("%2c",T->data);///T->data==(*T).data PreOrder(T->lchild);///先序遍历左子树 PreOrder(T->rchild);///先序遍历右子树 } } ///中序遍历输出 void InOrder(BiTree T) { if(T) { InOrder(T->rchild);///中序遍历右子树 printf("%2c",T->data); InOrder(T->lchild);///中序遍历左子树 } } ///后序遍历输出 void Postorder(BiTree T) { if(T) { Postorder(T->lchild);///后序遍历左子树 Postorder(T->rchild);///后序遍历右子树 printf("%2c",T->data);///访问根结点 } } ///层次遍历二叉树T,从第一层开始,每层从左到右 void LevleOrder(BiTree T) { BiTree Queue[MAX],b; ///用一维数组表示队列,front和rear分别表示队首和队尾指针 int front,rear; front=rear=0; if(T) ///若树非空 { Queue[rear++]=T;///根结点入队列 while(front!=rear) ///当队列非空 { b=Queue[front++];///队首元素出队列,并访问这个结点 printf("%2c",b->data); if(b->lchild!=NULL) { Queue[rear++]=b->lchild; ///左子树非空,则入队列 } if(b->rchild!=NULL) { Queue[rear++]=b->rchild; ///右子树非空,则入队列 } } } } ///求二叉树的深度 int depth(BiTree T){ int dep1,dep2; if(T==NULL) return 0; else { dep1=depth(T->lchild); dep2=depth(T->rchild); return dep1>dep2?dep1+1:dep2+1; } } int main() { BiTree T=NULL;///(开始定义的是*T)此时T为地址 printf("\n(Create a Binary Tree )建立一棵二叉树T:\n"); CreateBiTree(&T);///建立一棵二叉树 printf("\nThe preorder(先序序列为)is:\n"); PreOrder(T); printf("\nThe inorder(中序序列为)is:\n"); InOrder(T); printf("\nThe Postorder(后序序列为)is:\n"); Postorder(T); printf("\nThe levle order(层次序列为)is:\n"); LevleOrder(T); printf("\nThe depth(深度)is:%d\n",depth(T)); getchar(); return 0; }
1
2
3
4
5
6
7
8
9
10
11
12
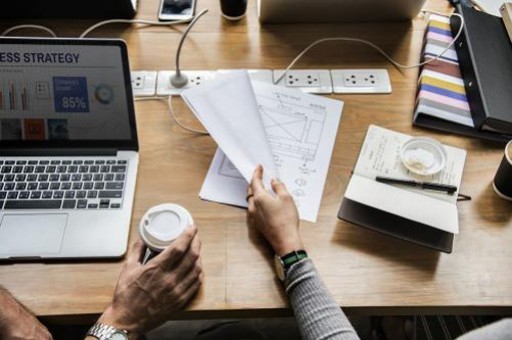
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
80
81
82
83
84
85
86
87
88
89
90
91
92
93
94
95
96
97
98
99
100
101
102
103
104
105
106
107
108
109
110
111
112
113
114
115
116
117
118
119
120
121
二叉树 数据结构
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。