宜收藏:5款常用的数据分析工具,简单便捷!数据分析工具常见的有哪些?
785
2022-05-30
# JavaWeb+MySQL实现简易留言板
> Hello,各位小伙伴们你们好,我是Bug终结者~,不知不觉,已经放假2周了,一晃来到了一周一更,今天我决定更新文章,今天为大家带来我学习过程中的一些经验,为小伙伴们避坑,下面为大家带来今日分享
## 留言板需求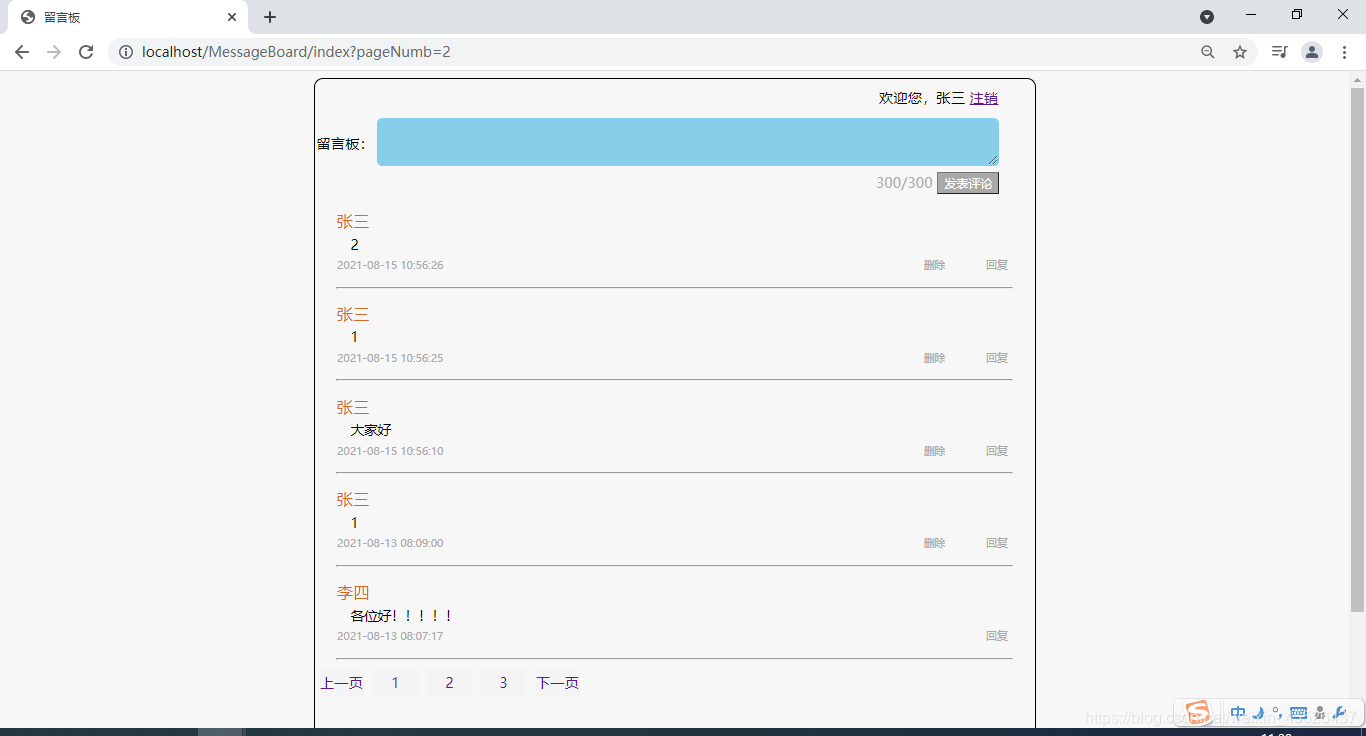
**上图显示的是评论页面的样式**
评论页面主要分为三部分: 登录状态区、 评论区(评论和回复操作的区域)、评论列表区(显示所有的评论、回复,以及翻页控件)
1. 登录状态区:
1.1 用户不登录也可以看到该列表,但必须登录才可以发表评论和回复。
1.2 用户不登录也可以看到评论区
1.3 用户不登录也可以看到回复按钮
1.4 用户未登录状态,在评论区上方显示: 注册 和 登录 超级链接,点击分别可以进入注册和登录页面。
1.5 登录状态,在评论区上方显示: 欢迎 XXXX ,注销 。
1.6 注销是超级链接,点击注销进入未登录状态。
2. 评论区:
2.1 默认显示为发表评论状态。
2.2 发表评论状态下,只显示 textarea控件 和 发表评论按钮。
点击某条评论的回复按钮,状态切换为针对该条评论的回复状态。
2.3 回复状态下,在评论区 textarea 上方将显示:
我要回复 XXX 在 2021-08-09 19:23 的评论: 听的又123
2.4 回复状态下,发表评论按钮,变成 发表回复
2.5 回复状态下,发表评论按钮右侧显示一个按钮“返回评论状态” ,点击将进入评论状态。
即评论区有两个状态: 评论状态和 回复状态
2.6 评论状态,发表的是 评论,回复状态是对某条评论回复评论。
2.7 回复状态是针对某条评论的。
3. 评论列表:
显示所有评论的列表。
显示评论人、评论时间、评论内容。
每条评论的右侧,有回复按钮,点击回复将进入针对该条评论的 回复状态。
登录状态下自己发表的评论,右侧有删除按钮
其他人发表的评论,右侧看不到删除按钮。
如果评论有回复,则在评论下显示回复列表。
每条回复显示 回复人、回复时间、回复内容。
在登录状态下,当前登录人可以看到自己回复记录的右侧有删除按钮。
评论要实现分页,不需要查询。
**看下效果:**
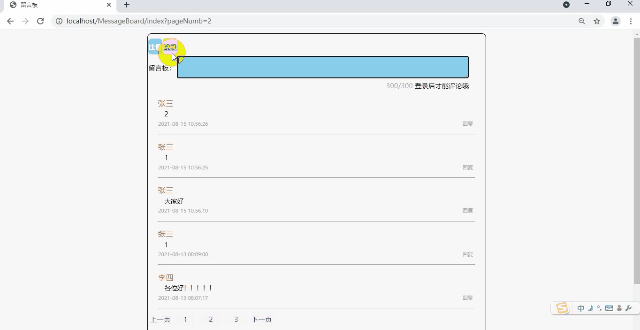
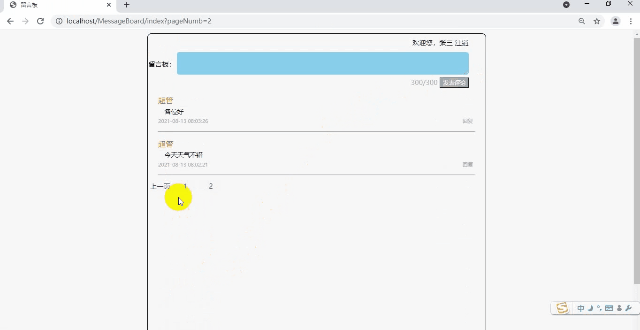
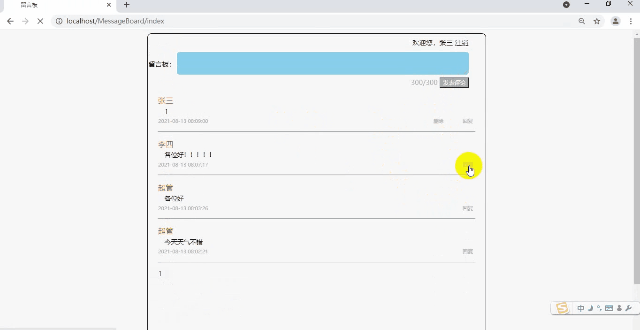
## 数据表
用户表
```sql
CREATE TABLE `t_user` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`account` varchar(32) COLLATE utf8mb4_unicode_ci NOT NULL,
`password` varchar(32) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
`realname` varchar(32) COLLATE utf8mb4_unicode_ci DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=14 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
```
评论表
```sql
CREATE TABLE `t_comment` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`user_id` int(11) NOT NULL COMMENT '评论人id,对应用户表的id',
`pl_content` varchar(500) COLLATE utf8mb4_unicode_ci NOT NULL COMMENT '评论的内容',
`pl_time` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP,
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=55 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
```
回复表
```sql
CREATE TABLE `t_revert` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`pl_id` int(11) NOT NULL COMMENT '评论人id,对应t_comment.id',
`user_id` int(11) DEFAULT NULL COMMENT '回复人id,对应当前登录的账号.id',
`hf_content` varchar(500) COLLATE utf8mb4_unicode_ci NOT NULL COMMENT '回复的内容',
`hf_time` timestamp NOT NULL DEFAULT CURRENT_TIMESTAMP ON UPDATE CURRENT_TIMESTAMP COMMENT '回复的时间',
PRIMARY KEY (`id`)
) ENGINE=InnoDB AUTO_INCREMENT=28 DEFAULT CHARSET=utf8mb4 COLLATE=utf8mb4_unicode_ci
```
**项目结构**
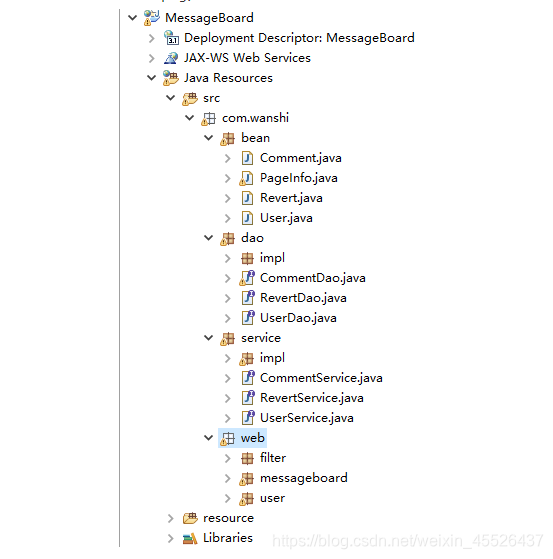
## 部分代码
**IndexServlet**
```java
@WebServlet("/index")
public class IndexServlet extends HttpServlet{
public static final Integer pageSize = 5;
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//获取当前页数
String strPageNumb = req.getParameter("pageNumb");
Integer pageNumb = 1;
if (!StringUtils.isEmpty(strPageNumb)) {
pageNumb = Integer.valueOf(strPageNumb);
}
CommentService commentService = new CommentServiceImpl();
try {
PageInfo pager = commentService.page(pageNumb, pageSize);
req.setAttribute("pager", pager);
req.getRequestDispatcher("/WEB-INF/comment.jsp")
.forward(req, resp);
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
```
**CommentServlet**
```java
@WebServlet("/comment")
public class CommentServlet extends HttpServlet{
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//获取数据,当前评论人的id,评论的内容
String content = req.getParameter("content");
User user = (User)req.getSession().getAttribute("user");
Integer userId = user.getId();
CommentService commentService = new CommentServiceImpl();
try {
if (commentService.saveComment(userId, content)) {
resp.sendRedirect(req.getContextPath()+"/index");
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
```
**RevertServlet**
```java
@WebServlet("/revert")
public class RevertServlet extends HttpServlet{
RevertService RevertService = new RevertServiceImpl();
CommentService CommentService = new CommentServiceImpl();
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//获取回复的记录id
String pl_id = req.getParameter("pl_id");
try {
Comment comment = CommentService.queryById(Integer.valueOf(pl_id));
System.out.println("123");
req.setAttribute("comment", comment);
req.getRequestDispatcher("/WEB-INF/revert.jsp")
.forward(req, resp);
} catch (NumberFormatException e) {
// TODO Auto-generated catch block
e.printStackTrace();
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
@Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
//获取回复的具体信息
String plId = req.getParameter("pl_id");
String liuYan = req.getParameter("liuYan");
System.out.println(plId);
User user = (User) req.getSession().getAttribute("user");
Integer userId = user.getId();
try {
if (RevertService.saveRevert(plId, liuYan, userId)) {
resp.sendRedirect(req.getContextPath()+"/index");
}
} catch (Exception e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
}
```
**Dao层核心代码 **
------
**连接数据库基类**
**BaseDao**
```java
public class BaseDao {
private static DataSource ds = null;
public QueryRunner initQueryRunner() throws Exception {
String dbFile = this.getClass().getClassLoader().getResource("/").getFile();
dbFile = dbFile.substring(1) + "db.properties";
FileReader fr = new FileReader(dbFile);
Properties pro = new Properties();
pro.load(fr);
// DataSource ds = DruidDataSourceFactory.createDataSource(pro);
if (ds == null) {
ds = DruidDataSourceFactory.createDataSource(pro);
}
QueryRunner qur = new QueryRunner(ds);
System.out.println(ds);
return qur;
}
}
```
**CommentDaoImpl**
```java
public class CommentDaoImpl extends BaseDao implements CommentDao{
@Override
public List
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "select * from t_comment order by pl_time desc limit ?, ? ";
return qur.query(sql, new BeanListHandler
}
@Override
public Comment queryById(Integer id) throws Exception {
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "select * from t_comment where id = ?";
return qur.query(sql, new BeanHandler
}
@Override
public Integer delete(String pl_id) throws Exception {
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "delete from t_comment where id = ?";
return qur.update(sql, pl_id);
}
@Override
public Integer saveComment(Integer userId, String content) throws Exception {
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "insert into t_comment (user_id, pl_content) values(?, ?)";
//如果update方法返回的大于0,代表增加成功,返回的小于0代表失败
return qur.update(sql, userId, content);
}
@Override
public Integer getCount() throws Exception {
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "select count(1) from t_comment";
Long rowCount = qur.query(sql, new ScalarHandler
return rowCount.intValue();
}
}
```
**RevertDaoImpl**
```jav
public class RevertDaoImpl extends BaseDao implements RevertDao{
@Override
public Integer saveRevert(String plId, String liuYan, Integer userId) throws Exception {
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "insert into t_revert (pl_id, user_id, hf_content) values(?, ?, ?)";
System.out.println(plId);
return qur.update(sql, plId, userId, liuYan);
}
@Override
public List
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "select * from t_revert where pl_id = ?";
return qur.query(sql, new BeanListHandler
}
@Override
public Integer delete(String hfId) throws Exception {
// TODO Auto-generated method stub
QueryRunner qur = initQueryRunner();
String sql = "delete from t_revert where id = ?";
return qur.update(sql, hfId);
}
}
```
## 絮语
> 今日经验分享到此就要结束了,代码路漫漫,有人与你共赴前行,遇到困难去解决,最后都会化为你宝贵的经验,每周一更,回忆一周内值得写的项目,把代码共享,分享自己做练习的一些思路,好了,本周分享该案例,我们下周见!
**都看到这了,点个赞支持一下博主吧~**
Java MySQL web前端
版权声明:本文内容由网络用户投稿,版权归原作者所有,本站不拥有其著作权,亦不承担相应法律责任。如果您发现本站中有涉嫌抄袭或描述失实的内容,请联系我们jiasou666@gmail.com 处理,核实后本网站将在24小时内删除侵权内容。